ACADEMIC RESEARCH AND PUBLICATIONS
during Graduate and Undergraduate Studies

Doctoral Candidate in Computer Science
Visual Computing Division, Digital Production Arts
Clemson University | School of Computing
LABRADORITE STANFORD BUNNY:
A COMPUTER GRAPHICS PROJECT
void loadTexture(char *filename, unsigned int texID)
{
FILE *fopen(), *fptr;
char buf[512];
int imgSize, imgWidth, imgHeight, maxColor, face;
unsigned char *texBytes, *parse;
fptr=fopen(filename,"r");
fgets(buf,512,fptr); //Strip first line.
do //Strip off comments.
{ fgets(buf,512,fptr); }
while(buf[0]=='#');
parse = strtok(buf," \t");
imgWidth = atoi(parse);
parse = strtok(NULL," \n");
imgHeight = atoi(parse);
fgets(buf,512,fptr);
parse = strtok(buf," \n");
maxColor = atoi(parse);
imgSize = imgWidth*imgHeight;
texBytes = (unsigned char *)calloc(3,imgSize);
fread(texBytes,3,imgSize,fptr);
fclose(fptr);
//Cube map to surround the object. Texture coords come from intersecting obj normals with a cube face.
glBindTexture(GL_TEXTURE_CUBE_MAP, texID);
for(face=0; face<6; face++)
{ //Each face of the cube map is a separate 2D image, indexing starting with the positive X face.
glTexImage2D(GL_TEXTURE_CUBE_MAP_POSITIVE_X + face,
0, GL_RGB, imgWidth, imgHeight, 0, GL_RGB,
GL_UNSIGNED_BYTE, texBytes);
}
glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_MAG_FILTER, GL_LINEAR); //Texture magnification with small (>=1) area of texture element.
glTexParameteri(GL_TEXTURE_CUBE_MAP, GL_TEXTURE_MIN_FILTER, GL_LINEAR);
free(texBytes);
}
<Vertex Shader>
varying vec3 eyeCoord_vNormal, eyeCoord_vPos; //Interpolated normals and positions between vertices
out vec3 texCoords; //Generate texture coordinates here to pass to the frag shader.
void main()
{
eyeCoord_vNormal = gl_NormalMatrix * gl_Normal; //gl_NormalMatrix OpenGL takes from the ModelView mat.
eyeCoord_vPos = gl_ModelViewMatrix * gl_Vertex;
texCoords = reflect(-eyeCoord_vPos, normalize(eyeCoord_vNormal)); //GLSL built-in function reflect calculates reflection vector using the eye direction (- vertex pos) and surface normal.
gl_Position = gl_ProjectionMatrix*gl_ModelViewMatrix*gl_Vertex; //gl_Position is necessary output of the vert shader.
}
Language: C (using OpenGL)
Date: Spring 2017
Class: Computer Graphics (Graduate)
This project entailed parsing a PLY geometry file and passing the information to OpenGL and GLSL for rendering. Features implemented are Blinn-Phong shading, 3-point lighting, and texture and environment mapping. The images used for the mapping are my own: clouds for the environment and labradorite for the bunny surface. The code provided is a sample of the project, on a texture loading function and the vertex shader.
Click on image to expand.
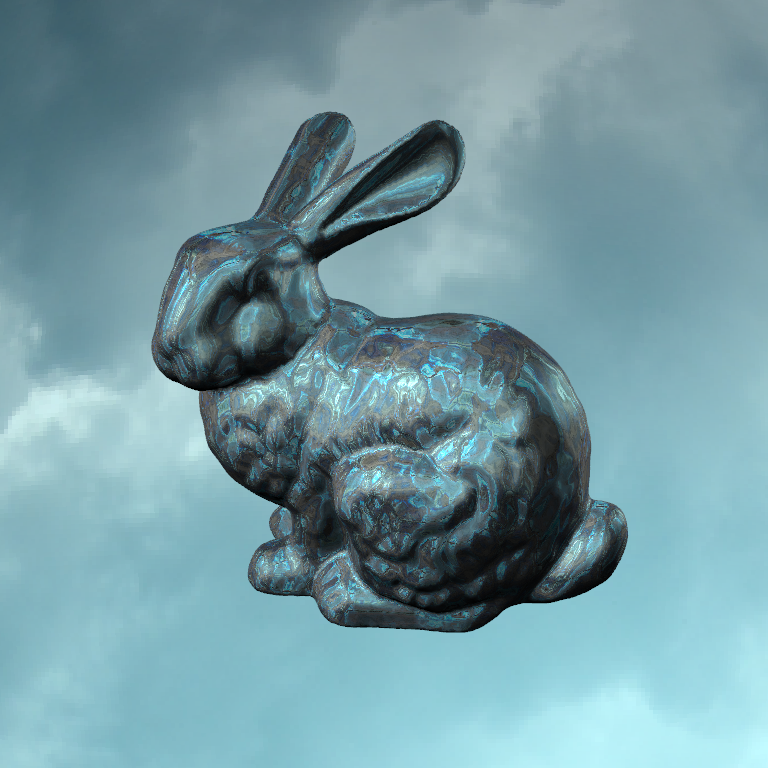
A window capture of the project.
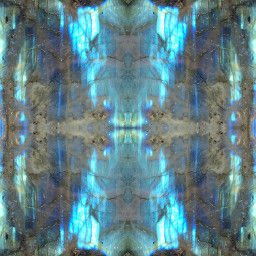
Photo I had taken of labradorite stone and edited for use as a tiled texture.